How to Work with Absolute Path Imports in Gatsby
While working on a Gatsby project, I found setting up absolute path imports slightly complicated. I prefer using the ~/
tilde notation for absolute imports over relative paths.
Example:
import Component from "~/components/Component"; // Absolute import
vs.
import Component from "../../../../components/Component"; // Relative import
Here’s how I made it work.
Adding a Custom Webpack Config
Gatsby provides plugins for this, but they often don’t work well with VS Code’s “Go to Definition.” Instead, you can configure Webpack manually in gatsby-node.js
:
const path = require("path");
exports.onCreateWebpackConfig = ({ actions }) => {
actions.setWebpackConfig({
resolve: {
alias: {
"~": path.resolve(__dirname, "src/"),
},
extensions: [".ts", ".tsx", ".js", ".jsx"],
},
});
};
Now, you can import files like this:
import Header from "~/components/layout/Header";
Issue: VS Code “Go to Definition” Doesn’t Work
Even with Webpack aliasing, VS Code may not recognize the paths, making navigation difficult.
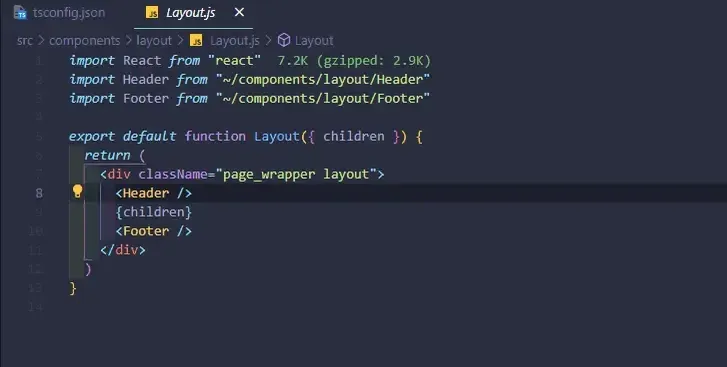
Fixing VS Code Path Resolution
To fix this, add a tsconfig.json
(for TypeScript) or jsconfig.json
(for JavaScript) in the root of your project:
{
"compilerOptions": {
"baseUrl": "./",
"paths": {
"~/*": ["src/*"]
}
}
}
Result: VS Code “Go to Definition” Now Works
With this setup, pressing Ctrl + Click
on a component or function will navigate to the correct file.
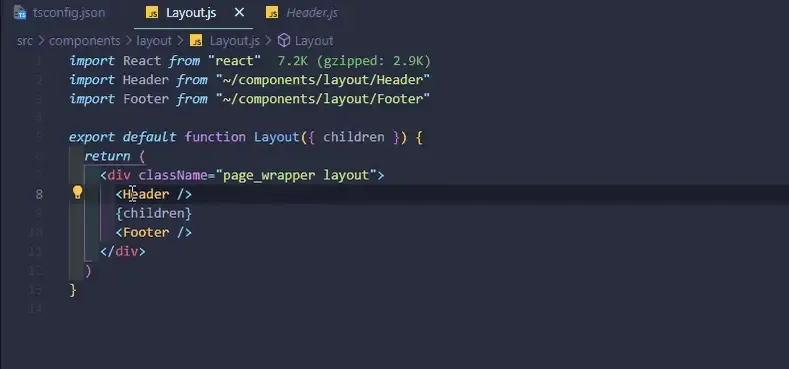
Feel free to reach out if you have any questions!