Create a flip card using html css and javascript
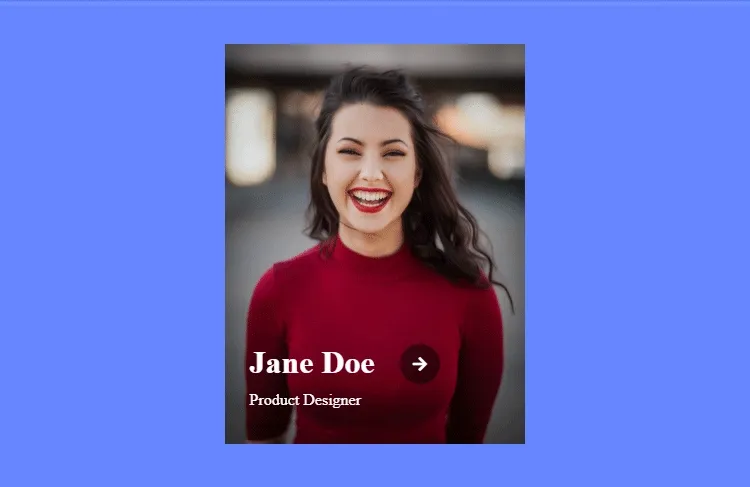
In today’s blog, I’ll show you how to create a simple flip card using HTML, CSS, and a few lines of JavaScript.
Flip cards are quite popular in modern web design. Understanding the core concept behind them allows you to create various interactive UI components using minimal code.
The Core Concept
How can we toggle between two classes on a button click? Imagine having two same-sized cards with different content—one should be visible while the other remains hidden. When a user clicks a button, the first card should be hidden, and the second card should appear.
The Solution
JavaScript provides a simple way to toggle visibility using CSS class manipulation. We can define a .hidden
class with display: none;
, and then dynamically add or remove it based on user interactions.
Code Implementation
1. Select Elements
We first need to select both card containers and the buttons using their respective IDs.
var rightArrow = document.getElementById("arrow_button");
var topCard = document.getElementById("cover_image");
var bottomCard = document.getElementById("detail_content");
var followButton = document.getElementById("follow_button");
2. Add Event Listeners
We’ll attach event listeners to the buttons and toggle the .hidden
class accordingly.
rightArrow.addEventListener("click", function () {
topCard.classList.add("hidden");
bottomCard.classList.remove("hidden");
console.log("Hiding top card and showing bottom card.");
});
followButton.addEventListener("click", function () {
topCard.classList.remove("hidden");
bottomCard.classList.add("hidden");
console.log("Hiding bottom card and showing top card.");
});
Final Thoughts
That’s it! With just a few lines of JavaScript, we’ve built a simple flip card. More importantly, you now understand how to dynamically add and remove classes using JavaScript, which can be applied to many other interactive UI elements.
Stay safe and happy coding! 🙂